Google Colab GPU is a free cloud service offered by Google that allows users to run their code on a remote GPU. This service is available to all users, and no setup is required. It is primarily used for interactive use cases and provides users with access to more reliable resources than other free cloud services. To use the free GPU provided by Google in Colab notebooks, users must select the “Runtime / Change runtime type” menu option and select “GPU.”
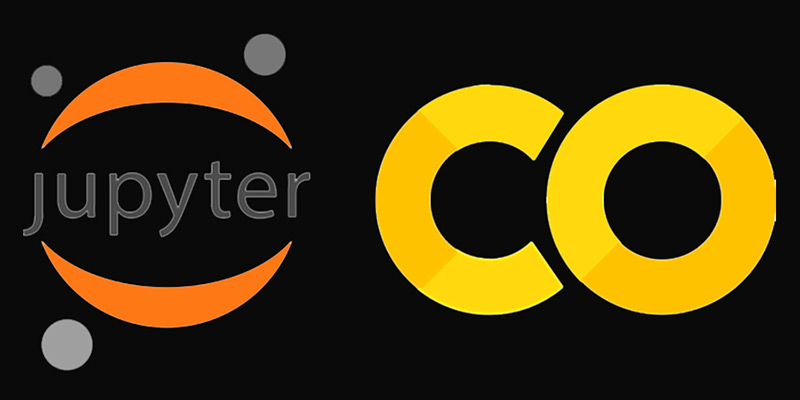
Once the GPU is enabled, users can use the following function to test if it is enabled and the “!cat /proc/meminfo” command to see the memory resources available for their process.
import tensorflow as tf
tf.config.list_physical_devices('GPU')
After this, the notebook will automatically recognize and use the GPU for any computations that require it. Furthermore, it is also possible to configure the GPU memory growth with the following code snippet:
gpus = tf.config.experimental.list_physical_devices('GPU')
if gpus:
try:
for gpu in gpus:
tf.config.experimental.set_memory_growth(gpu, True)
logical_gpus = tf.config.experimental.list_logical_devices('GPU')
print(len(gpus), "Physical GPUs,", len(logical_gpus), "Logical GPUs")
except RuntimeError as e:
print(e)
GPU Specifications
Google Colab GPUs provide users with a powerful machine learning environment. They feature an NVIDIA Tesla K80 GPU, two vCPUs @ 2.2GHz, 12GB of RAM, and 100GB of free space. Additionally, they have an idle cut-off of 90 minutes and a maximum runtime of 12 hours. With these specs, Colab GPUs are capable of running complex machine learning tasks quickly and efficiently.
What It Can Do
Colab GPUs can be used for a variety of complex tasks, such as training and deploying deep learning models, natural language processing, image processing and analysis, audio processing, and more. With the powerful specs provided, it can easily handle the demands of these tasks and provide users with a reliable and performing environment for their projects. As such, Colab GPUs are a great choice for developers and researchers who need to quickly and efficiently finish their projects.
Use Case Example
The following code snippet shows how to use a Colab GPU to train a TensorFlow model using sample input from a Machine Learning dataset:
"""
The Fashion-MNIST dataset consists of 60,000 28x28 grayscale images of 10 classes, such as shirts, trousers, shoes, and handbags.
"""
mnist = tf.keras.datasets.mnist
(x_train, y_train), (x_test, y_test) = mnist.load_data()
x_train, x_test = x_train / 255.0, x_test / 255.0
model = tf.keras.models.Sequential([
tf.keras.layers.Flatten(input_shape=(28, 28)),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dropout(0.2),
tf.keras.layers.Dense(10)
])
"""
The variable predictions is assigned the output of a function call to model() with x_train[:1] as an argument. The .numpy() method is used to convert the prediction from a TensorFlow type to a NumPy array which holds numerical values.
"""
predictions = model(x_train[:1]).numpy()
"""
The function takes an array of numerical values and returns an array of the same size, with each element representing a probability between 0 and 1. The .numpy() method is used to convert the output from a TensorFlow type to a NumPy array. This output can then be used to interpret the probabilities of each prediction.
"""
tf.nn.softmax(predictions).numpy()
"""
The SparseCategoricalCrossentropy function is a loss function used to calculate the difference between a predicted output and the actual output. The from_logits argument is set to True, which means that the output of the model is expected to be in a logit form. This means that the output of the model must be a probability between 0 and 1 and the logit of this probability must be taken before calculating the loss.
"""
loss_fn = tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True)
"""
The method to configure a machine learning model for training. The optimizer argument is set to 'adam', which specifies the Adam optimizer. The loss argument is set to the previously defined loss_fn, which is a SparseCategoricalCrossentropy loss function. The metrics argument is set to ['accuracy'], which specifies that the accuracy metric will be used when evaluating the model's performance.
"""
model.compile(optimizer='adam',
loss=loss_fn,
metrics=['accuracy'])
# Check GPU availability
gpus = tf.config.experimental.list_physical_devices('GPU')
if gpus:
try:
for gpu in gpus:
tf.config.experimental.set_memory_growth(gpu, True)
logical_gpus = tf.config.experimental.list_logical_devices('GPU')
print(len(gpus), "Physical GPUs,", len(logical_gpus), "Logical GPUs")
except RuntimeError as e:
print(e)
"""
We call fit(), which will train the model by slicing the data into "batches" of size batch_size, and repeatedly iterating over the entire dataset for a given number of epochs.
"""
model.fit(x_train, y_train, epochs=5)
"""
The method to evaluate performance of a machine learning model. The x_test and y_test arguments are used to specify the test data that will be used to evaluate the model. The verbose argument is set to 2, which specifies that the evaluation process should be silent and only the final evaluation result should be printed out.
"""
model.evaluate(x_test, y_test, verbose=2)
Google Colab FAQs
There are several Frequently Asked Questions (FAQs) related to Google Colab. These include questions such as how to create a Colab Notebook, how to use a Colab GPU, and how to import data into Colab. Additionally, there are questions about how to upload files or save Colab notebooks, how to use Colab with Jupyter, and how to access Colab files offline. Furthermore, there are also questions about how to run code quickly in Colab, how to manage and debug Colab notebooks, and how to access additional Colab features. [1] For more detailed information on Colab FAQs, visit the Colaboratory FAQs page. [2]
References:
[1] Google Colab
[2] About Colab – Colab Help – Google Support
[3] Google Colab: Everything you Need to Know – Geekflare
Conclusion
Google Colab is an incredibly powerful platform for data scientists and researchers. It allows them to easily access datasets and build models. To learn more about Google Colab, you can have a look at the blog post [1] which provides an exhaustive overview of its features. Additionally, you can also learn how to download datasets from Kaggle [2] and explore some of the basic differences between Google Colab and other cloud services [3].
References:
[1] 10 tricks for a better Google Colab experience
[2] How to Deal With Files in Google Colab: Everything You Need …
[3] Reading and Writing files with Google Colaboratory – Medium