There are several popular Python libraries for creating graphical user interfaces (GUIs):
- Tkinter: Tkinter is a standard Python library and is included in most Python distributions. It is built on top of the Tk toolkit and is easy to learn and use. Tkinter is cross-platform and suitable for both beginners and experienced developers alike.
- PyQt: PyQt is a set of Python bindings for the Qt application development framework. Qt is a popular library for creating cross-platform graphical user interfaces. PyQt is a powerful library and is suitable for creating complex and sophisticated GUIs.
- wxPython: wxPython is a wrapper for the popular wxWidgets library. It provides an easy-to-use and powerful set of tools for creating GUIs. wxPython is a good choice for developers looking for a simple and powerful library for creating graphical applications.
- Kivy: Kivy is an open source Python library for creating modern, cross-platform graphical user interfaces. It is based on OpenGL ES and provides a powerful framework for developing multi-platform applications. Kivy is suitable for creating both simple and complex GUIs.
Create a small GUI using Tkinter
Tkinter is a standard Python library for creating graphical user interfaces (GUIs). It is built on top of the Tk toolkit, which is a set of widgets that helps developers create windows and graphical elements in an application. With Tkinter, developers can create applications that have a graphical user interface. It is easy to learn and is suitable for both beginners and experienced developers alike. Tkinter is also cross-platform, meaning that applications written using it can be run on multiple operating systems without needing to be ported or rewritten.
Tkinter is a versatile library, and is used for a variety of applications. Some popular uses for Tkinter include:
- Creating desktop applications
- Building graphical user interfaces
- Designing games with a graphical interface
- Creating software for scientific and mathematical simulations
- Developing tools for data visualization
- Developing custom widgets for the desktop
- Creating graphical user interfaces for the web
- Creating graphical user interfaces for mobile devices
In this example, we use the tkinter library to create a small graphical application. The app variable represents the main window, and we create a label and a button inside it. We then use the mainloop() method to start the application. Once the application is running, clicking on the button will print the message “You clicked me!” in the console. This is just a very basic example, but it illustrates how easy it is to create small graphical apps with tkinter.
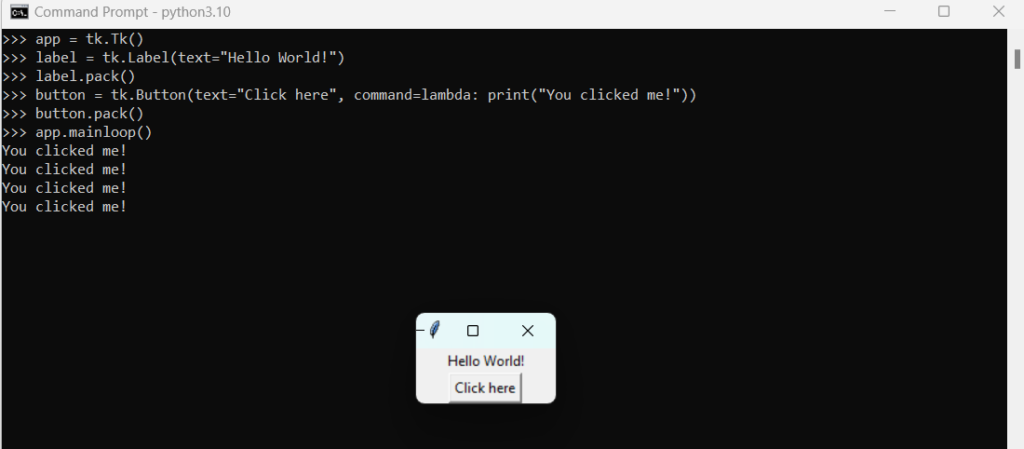
import tkinter as tk
app = tk.Tk()
# Create a label
label = tk.Label(text="Hello World!")
label.pack()
# Create a button
button = tk.Button(text="Click here", command=lambda: print("You clicked me!"))
button.pack()
# Start the application
app.mainloop()
Another example creates a graphical user interface (GUI) that can be used to visualize data. The code starts by importing the Tkinter library, which enables the user to create a window and frame. Next, a canvas is created, and the graph is plotted using the create_line() method. Finally, the window is displayed using the mainloop() method. This code allows the user to visualize data in an interactive and graphical way.
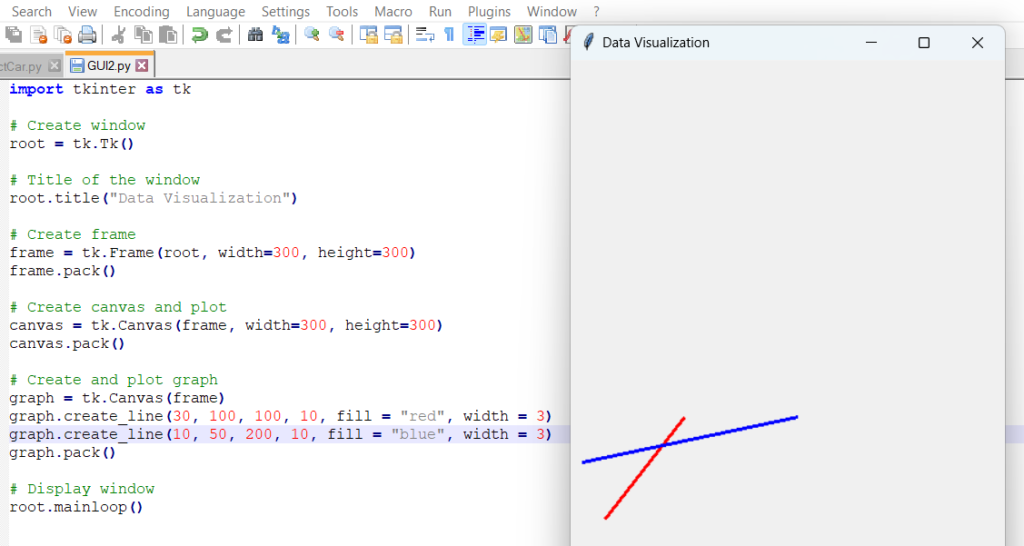
import tkinter as tk
# Create window
root = tk.Tk()
# Title of the window
root.title("Data Visualization")
# Create frame
frame = tk.Frame(root, width=300, height=300)
frame.pack()
# Create canvas and plot
canvas = tk.Canvas(frame, width=300, height=300)
canvas.pack()
# Create and plot graph
graph = tk.Canvas(frame)
graph.create_line(30, 100, 100, 10, fill = "red", width = 3)
graph.create_line(10, 50, 200, 10, fill = "blue", width = 3)
graph.pack()
# Display window
root.mainloop()
Pingback: Intro to Python Programming for Kids – Deep Learning Malaysia