The Sequential model is a foundational building block of deep learning in Keras, an open-source software library for machine learning. It provides a simple and intuitive way to create deep neural networks, where layers are stacked sequentially on top of each other to form a pipeline of data transformations.
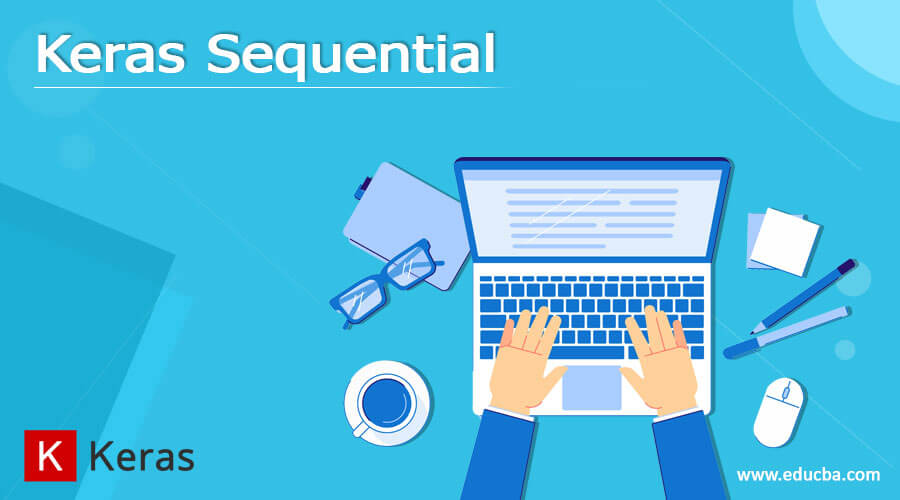
In a Sequential model, data flows through each layer in a fixed order, starting from the input layer and ending with the output layer. Each layer can perform a different type of transformation on the data, such as convolutions, pooling, dropout, or dense connections. By stacking these layers together, a Sequential model can learn complex patterns and representations from the data, making it a powerful tool for many different types of machine learning tasks.
The Sequential model in Keras is easy to use and highly customizable, with many different types of layers, activation functions, loss functions, and optimizers to choose from. It is also compatible with many different types of data, such as images, text, and time-series data. With its flexibility and power, the Sequential model has become a staple of modern deep learning and continues to be a popular choice for many different types of projects.
Keras Sequential Model
Here’s an example of a general Sequential model with hyperparameters that can be customized for various tasks:
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Dropout, Flatten, Conv2D, MaxPooling2D, LSTM, Embedding, SimpleRNN, GRU, BatchNormalization
from tensorflow.keras.optimizers import Adam, SGD, RMSprop, Adagrad, Adadelta, Ftrl, Nadam
from tensorflow.keras.losses import binary_crossentropy, categorical_crossentropy, mean_squared_error, mean_absolute_error, huber, logcosh, poisson, cosine_similarity
from tensorflow.keras.metrics import accuracy, binary_accuracy, categorical_accuracy, top_k_categorical_accuracy, sparse_categorical_accuracy, mean_io_u, precision
# Define the hyperparameters
num_classes = 10
input_shape = (32, 32, 3)
learning_rate = 0.001
dropout_rate = 0.2
activation = 'relu'
optimizer = Adam()
loss_function = categorical_crossentropy
metric = accuracy
# Create the model
model = Sequential()
# Add the layers
model.add(Conv2D(32, kernel_size=(3, 3), activation=activation, input_shape=input_shape))
model.add(Conv2D(64, (3, 3), activation=activation))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(dropout_rate))
model.add(Flatten())
model.add(Dense(128, activation=activation))
model.add(Dropout(dropout_rate))
model.add(Dense(num_classes, activation='softmax'))
# Compile the model
model.compile(optimizer=optimizer, loss=loss_function, metrics=[metric])
# Print the summary of the model
model.summary()
This Sequential model is suitable for an image classification task, where the input data consists of 2D images with color channels (e.g., RGB color images).
The first two layers are convolutional layers, where the first layer has 32 filters with a kernel size of (3, 3) and the second layer has 64 filters with the same kernel size. The activation function used in both layers is specified by the activation
variable.
The third layer is a max pooling layer with a pool size of (2, 2), which reduces the spatial dimensions of the output from the convolutional layers. The fourth layer is a dropout layer, which randomly drops out a fraction of the neurons in the previous layer to prevent overfitting.
The fifth layer is a flatten layer, which converts the output of the previous layer into a one-dimensional array that can be used as input to a fully connected layer. The sixth and seventh layers are dense layers, where the first dense layer has 128 neurons and the second dense layer has num_classes
neurons. Both dense layers use the specified activation
function.
The final output layer has a softmax
activation, which is suitable for multi-class classification tasks. Overall, this model is suitable for tasks such as image classification or object recognition, where the goal is to classify images into one of num_classes
categories. The model can be customized by adjusting the hyperparameters (e.g., changing the number of filters or neurons in the dense layers, or adjusting the dropout rate) to optimize its performance for a specific task.
Keras API reference
Keras Layers
Here’s a list of common layers available in Keras and their typical uses:
Dense
: This layer is a fully connected layer, where every neuron in the layer is connected to every neuron in the previous layer. It is one of the most common layers in a neural network and can be used for both classification and regression tasks.Conv2D
: This layer is a 2D convolutional layer, which is commonly used in image processing and computer vision tasks. It applies a set of filters to an input image to extract features.MaxPooling2D
: This layer is a 2D max pooling layer, which is commonly used in combination with Conv2D layers to reduce the size of the feature maps and capture the most important features.Flatten
: This layer flattens the output of the previous layer into a one-dimensional array, which can be used as input to a fully connected layer.Dropout
: This layer randomly drops out a fraction of the neurons in the previous layer during training, which can help prevent overfitting.BatchNormalization
: This layer normalizes the activations of the previous layer to have zero mean and unit variance, which can help prevent overfitting and improve the stability of the model.LSTM
: This layer is a type of recurrent neural network (RNN) layer that is commonly used in natural language processing tasks. It is capable of processing sequences of inputs and has a memory mechanism that allows it to retain information from previous inputs.GRU
: This layer is another type of recurrent neural network (RNN) layer that is similar to the LSTM layer, but has fewer parameters and is often faster to train.Embedding
: This layer is commonly used in natural language processing tasks to map each word in a vocabulary to a vector representation. It is often used as the first layer in a neural network for text data.SimpleRNN
: This layer is a simple type of recurrent neural network layer that can be used for tasks that involve sequential data. It has a memory mechanism that allows it to retain information from previous inputs.
These are some of the common layers available in Keras, but there are also many others that are more specialized for specific tasks. When building a neural network, it’s important to select the appropriate layers for the task and to consider the properties of the data being used.
Keras Activation functions
Here’s a list of common activation functions available in Keras and their typical uses:
sigmoid
: This activation function is commonly used in the output layer of a binary classification model. It maps any input value to a probability between 0 and 1, which can be interpreted as the probability of the input belonging to a particular class.softmax
: This activation function is commonly used in the output layer of a multi-class classification model. It maps a vector of input values to a probability distribution over the classes, so that the sum of the probabilities is equal to 1.relu
or rectified linear unit: This activation function is commonly used in hidden layers of a neural network. It is a simple and computationally efficient function that maps any negative input value to 0, and any positive input value to itself. It has been found to work well in many applications and can help with the vanishing gradient problem.tanh
or hyperbolic tangent: This activation function is similar tosigmoid
, but maps any input value to a range between -1 and 1. It is commonly used in hidden layers of a neural network, especially when the inputs are standardized.elu
or exponential linear unit: This activation function is a variant ofrelu
that maps negative input values to a small negative value instead of 0. It has been found to work well in some applications and can help with the vanishing gradient problem.selu
or scaled exponential linear unit: This activation function is a self-normalizing variant ofelu
that maintains the mean and variance of the input values. It has been found to work well in deep neural networks.softplus
: This activation function is a smoothed version of therelu
function, and is often used in applications where the model needs to output positive values.swish
: This activation function is a relatively new function that has been found to work well in some applications. It is a smooth function that maps any input value to a range between 0 and the input value itself.
These are some of the common activation functions available in Keras, but there are also many others that are more specialized for specific tasks. When selecting an activation function, it’s important to consider the type of task, the properties of the data, and the properties of the model being trained.
Keras Loss functions
Here’s a list of common loss functions available in Keras and their typical uses:
binary_crossentropy
: This loss function is used for binary classification tasks, where there are only two possible classes. It measures the cross-entropy between the true labels and predicted probabilities.categorical_crossentropy
: This loss function is used for multi-class classification tasks, where there are more than two possible classes. It measures the cross-entropy between the true labels and predicted probabilities.sparse_categorical_crossentropy
: This loss function is similar tocategorical_crossentropy
, but is used when the true labels are integers (e.g., in a classification task where the labels are 0, 1, 2, etc.). It is more memory-efficient thancategorical_crossentropy
when there are many classes.mse
ormean_squared_error
: This loss function is used for regression tasks, where the goal is to predict a continuous numeric value. It measures the mean squared difference between the true values and predicted values.mae
ormean_absolute_error
: This loss function is also used for regression tasks. It measures the mean absolute difference between the true values and predicted values.huber
: This loss function is a combination ofmse
andmae
. It is less sensitive to outliers thanmse
and provides a smoother gradient thanmae
.logcosh
: This loss function is similar tohuber
and is also less sensitive to outliers. It is a smoothed version of the log(cos(x)) function.poisson
: This loss function is used when the true values are counts (e.g., in a prediction task for the number of events in a certain period). It measures the difference between the true counts and predicted counts based on the Poisson distribution.cosine_similarity
: This loss function measures the cosine similarity between the true values and predicted values. It is often used in tasks such as image retrieval, where the goal is to find similar images based on their features.
These are some of the common loss functions available in Keras, but there are also many others that are more specialized for specific tasks. When selecting a loss function, it’s important to consider the type of task, the nature of the data, and the properties of the model being trained.
Keras Optimizers
Here’s a list of common optimizers available in Keras and their typical uses:
SGD
or Stochastic Gradient Descent: This optimizer is a simple and commonly used method for updating the weights of a neural network during training. It updates the weights based on the gradient of the loss function with respect to the weights, multiplied by a learning rate.RMSprop
: This optimizer is a variant of stochastic gradient descent that divides the learning rate by a moving average of the magnitudes of the gradients. It can help prevent the learning rate from getting too large or too small.Adam
: This optimizer is a popular variant of stochastic gradient descent that combines ideas from RMSprop and momentum. It maintains a moving average of the gradients and the squared gradients, and adjusts the learning rate adaptively.Adagrad
: This optimizer adapts the learning rate for each parameter based on the historical gradient information for that parameter. It is well-suited for sparse data and can help prevent the learning rate from getting too large.Adadelta
: This optimizer is a variant of Adagrad that maintains a moving average of the gradients and the squared parameter updates. It adjusts the learning rate adaptively and can help prevent the learning rate from getting too small.Nadam
: This optimizer is a variant of Adam that incorporates Nesterov momentum, which can help accelerate convergence.Ftrl
: This optimizer is designed for sparse data and uses the FTRL-Proximal algorithm to update the weights. It can help prevent overfitting and is well-suited for large-scale problems.Adamax
: This optimizer is a variant of Adam that uses the infinity norm of the gradients instead of the L2 norm. It can be more robust to noise and can work well in deep neural networks.
These are some of the common optimizers available in Keras, but there are also many others that are more specialized for specific tasks. When selecting an optimizer, it’s important to consider the type of task, the properties of the data, and the properties of the model being trained.
Keras Metrics
Here’s a list of common metrics available in Keras and their typical uses:
accuracy
: This metric is commonly used in classification tasks and measures the proportion of correctly classified samples.binary_accuracy
: This metric is a variant ofaccuracy
and is used in binary classification tasks.categorical_accuracy
: This metric is a variant ofaccuracy
and is used in multi-class classification tasks.top_k_categorical_accuracy
: This metric measures the proportion of samples where the true label is among the top k predicted labels. It is often used in multi-class classification tasks.sparse_categorical_accuracy
: This metric is a variant ofcategorical_accuracy
and is used when the true labels are integers (e.g., in a classification task where the labels are 0, 1, 2, etc.).mse
ormean_squared_error
: This metric is commonly used in regression tasks and measures the mean squared difference between the true values and predicted values.mae
ormean_absolute_error
: This metric is also used in regression tasks and measures the mean absolute difference between the true values and predicted values.cosine_similarity
: This metric measures the cosine similarity between the true values and predicted values. It is often used in tasks such as image retrieval, where the goal is to find similar images based on their features.mean_io_u
or mean Intersection over Union: This metric is commonly used in segmentation tasks and measures the overlap between the predicted segmentation and the true segmentation.precision
: This metric measures the proportion of true positive samples among all predicted positive samples. It is often used in binary classification tasks.
These are some of the common metrics available in Keras, but there are also many others that are more specialized for specific tasks. When selecting a metric, it’s important to consider the type of task, the properties of the data, and the properties of the model being trained.
Keras Callbacks
Keras provides several built-in callbacks that can be used to monitor the training of a deep learning model and modify its behavior as needed. Here’s a list of all the Keras callbacks and their functions:
- ModelCheckpoint: Save the model after every epoch or when a certain metric has improved.
- EarlyStopping: Stop training when a monitored metric has stopped improving for a specified number of epochs.
- TensorBoard: Log training metrics and visualize them using TensorBoard.
- ReduceLROnPlateau: Reduce the learning rate when a monitored metric has stopped improving.
- CSVLogger: Log the training metrics to a CSV file.
- TerminateOnNaN: Stop training when a NaN loss is encountered.
- LambdaCallback: Perform arbitrary actions at different stages of the training process.
- LearningRateScheduler: Dynamically adjust the learning rate based on the epoch or other criteria.
- RemoteMonitor: Monitor training using a remote server.
- ProgbarLogger: Display the training progress as a progress bar.
- History: Record the training metrics in a History object.
- BaseLogger: Record the training metrics in memory and print them at the end of the training.
- Callback: The base class for all Keras callbacks.
These built-in callbacks can be used in combination to create a customized training process that is tailored to your specific deep learning model and use case. To use a callback, you simply pass it to the fit()
method of your Keras model. For example, to use the ModelCheckpoint
callback to save the best model during training, you can use the following code:
from keras.callbacks import ModelCheckpoint
# define the callback
checkpoint = ModelCheckpoint(filepath='best_model.h5',
monitor='val_loss',
save_best_only=True)
# train the model with the callback
model.fit(x_train, y_train,
validation_data=(x_val, y_val),
epochs=10,
batch_size=32,
callbacks=[checkpoint])
This code will save the model to a file called best_model.h5
whenever the validation loss improves, so you can use the best model for prediction later on.
Keras Preprocessing
Keras provides a number of built-in pre-processing utilities that can be used to prepare data for machine learning models. Here’s a list of all the Keras pre-processing utilities:
- ImageDataGenerator: Generate batches of augmented image data with real-time data augmentation.
- Sequence: Generate batches of time-series data for input to a model.
- Tokenizer: Convert text into a sequence of integers.
- pad_sequences: Pad sequences to a specified length.
- normalize: Scale and normalize input data.
- to_categorical: Convert class vectors (integers) to binary class matrices.
- center_crop: Center crop an image to a specified size.
- smart_resize: Resize an image while preserving its aspect ratio and cropping to avoid distortion.
- random_crop: Randomly crop an image to a specified size.
- random_rotation: Randomly rotate an image.
- random_shift: Randomly shift an image.
- random_shear: Randomly shear an image.
- random_zoom: Randomly zoom an image.
- random_brightness: Randomly adjust the brightness of an image.
- random_contrast: Randomly adjust the contrast of an image.
- random_flip: Randomly flip an image horizontally or vertically.
These pre-processing utilities can be used in combination to create a customized data pre-processing pipeline that is tailored to your specific machine learning model and use case. To use a pre-processing utility, you simply call the function and pass in the data that you want to pre-process. For example, to use the to_categorical
utility to convert class labels to binary class matrices, you can use the following code:
from keras.utils import to_categorical
# convert class labels to binary class matrices
y_train = to_categorical(y_train, num_classes)
y_test = to_categorical(y_test, num_classes)
This code will convert the class labels to binary class matrices, which can be used as input to a Keras model for multi-class classification.
Keras Transfer Learning
Transfer learning is a technique in deep learning where a pre-trained model is used as a starting point for a new task, instead of training a new model from scratch. Keras provides several pre-trained models that can be used for transfer learning. Here’s a list of all the Keras pre-trained models for transfer learning:
- VGG16: A 16-layer convolutional neural network trained on the ImageNet dataset.
- VGG19: A 19-layer convolutional neural network trained on the ImageNet dataset.
- ResNet50: A 50-layer residual network trained on the ImageNet dataset.
- InceptionV3: A deep convolutional neural network with 48 layers trained on the ImageNet dataset.
- Xception: A deep convolutional neural network with 71 layers trained on the ImageNet dataset.
- MobileNet: A lightweight convolutional neural network trained on the ImageNet dataset.
- DenseNet: A deep convolutional neural network with dense connections trained on the ImageNet dataset.
- NASNet: A neural architecture search neural network trained on the ImageNet dataset.
These pre-trained models can be used as feature extractors, by removing the top layers of the model and adding new layers on top for the specific task at hand. Alternatively, they can be fine-tuned by unfreezing the top layers of the model and retraining the entire model on a new task.
To use a pre-trained model in Keras, you can import the model using the tensorflow.keras.applications
module. For example, to import the VGG16 model, you can use the following code:
from tensorflow.keras.applications.vgg16 import VGG16
# load the pre-trained VGG16 model
model = VGG16(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
This code will load the pre-trained VGG16 model and remove the top layers of the model. You can then add new layers on top of the model for the specific task at hand, or fine-tune the entire model for a new task.
Further readings
If you’re interested in learning more about the Keras API, here are some resources that you might find useful:
- Keras Documentation: The official documentation for Keras provides a comprehensive reference for all the Keras functions and classes. It includes tutorials, guides, and examples to help you get started with Keras.
- Keras Code Examples: The Keras code examples repository on GitHub contains a variety of code examples that demonstrate how to use Keras for different deep learning tasks, such as image classification, text classification, and time series prediction.
- Deep Learning with Python: The book “Deep Learning with Python” by Francois Chollet, the creator of Keras, provides an in-depth guide to deep learning with Keras. It covers a wide range of topics, from basic deep learning concepts to advanced techniques like generative adversarial networks (GANs).
- Keras Tuner: Keras Tuner is a hyperparameter tuning library for Keras that allows you to search for the best hyperparameters for your deep learning model. It includes a range of tuners, from random search to Bayesian optimization, and integrates with Keras seamlessly.
- TensorFlow Tutorials: TensorFlow provides a range of tutorials that demonstrate how to use Keras with TensorFlow for different deep learning tasks. These tutorials cover a wide range of topics, from image classification to natural language processing.
These resources should provide you with a good starting point for learning more about the Keras API and how to use it for your own deep learning projects.