Sequential data is a type of data that is ordered in a specific way, such that the order of the data points matters. In other words, the information in the data is presented in a sequence or a series, where each data point is related to the one that came before it and/or the one that comes after it. Examples of sequential data include time series data, where the data points are recorded over time, or natural language, where the words in a sentence are presented in a specific order and are related to each other in some way.
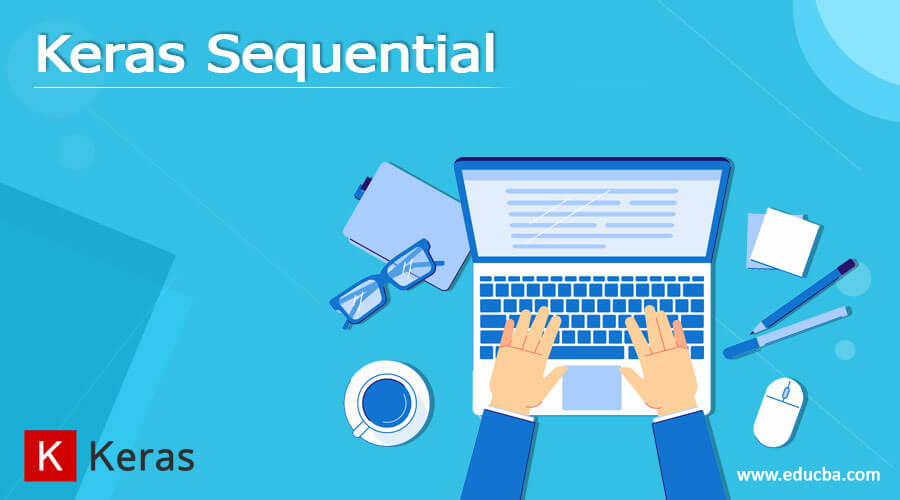
Sequential data presents a unique challenge for machine learning algorithms, because the order of the data points matters, and the context of each data point depends on the data points that came before it. This means that traditional machine learning techniques, such as regression or classification, may not be suitable for analyzing sequential data.
Sequential data is ubiquitous in many fields, including finance, weather forecasting, speech recognition, and natural language processing, and is an important area of research in machine learning and artificial intelligence.
Facts about RNNs, GRUs, and LSTMs
One interesting aspect about RNNs, GRUs, and LSTMs is that they are all types of neural networks that are specifically designed to handle sequential data, such as time series or natural language. These networks have a unique ability to maintain a form of memory or context, which allows them to make predictions or decisions based on the information they have processed so far.
For example, in the case of a language model that predicts the next word in a sentence, the RNN, GRU, or LSTM can use the previous words in the sentence as context to make a more accurate prediction. The network can learn to recognize patterns and dependencies in the data, such as subject-verb agreement or noun-adjective pairs, and use this information to generate more meaningful and coherent predictions.
Another interesting aspect of these networks is their use of gating mechanisms, which allow the network to control the flow of information and selectively remember or forget certain pieces of information. The GRU and LSTM, in particular, use sophisticated gating mechanisms to selectively update their internal state based on the input data and the current state. This allows them to handle long-term dependencies in the data and avoid the vanishing gradient problem that can occur in traditional RNNs.
Overall, RNNs, GRUs, and LSTMs represent an important class of neural networks that are well-suited for handling sequential data, and have a range of interesting applications in natural language processing, speech recognition, and other domains. Their unique ability to maintain context and handle long-term dependencies make them a powerful tool for modeling and predicting complex patterns in sequential data.
TensorFlow Sequential model
The Sequential
model is a high-level API in TensorFlow that allows you to create and train neural networks with ease. The Sequential
model is a linear stack of layers, where you can add layers one by one to build the neural network. This makes it easy to create a variety of neural network architectures without having to manually connect the layers together.
To create a Sequential
model in TensorFlow, you start by instantiating a Sequential
object:
from tensorflow.keras.models import Sequential
model = Sequential()
Once you have created the Sequential
object, you can add layers to it using the add
method. For example, to add a dense layer with 32 units and a ReLU activation function, you would use:
from tensorflow.keras.layers import Dense, Activation
model.add(Dense(32))
model.add(Activation('relu'))
You can add as many layers as you need, and customize the type of layer, number of units, activation function, and other parameters as required.
After adding the layers, you need to compile the model by specifying a loss function, an optimizer, and optionally some metrics to track during training. For example, to compile the model with a binary cross-entropy loss function and the Adam optimizer, you would use:
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
Finally, you can train the model on your data using the fit
method, which takes in the input data, target data, and other parameters such as the batch size and number of epochs:
model.fit(x_train, y_train, batch_size=32, epochs=10, validation_data=(x_val, y_val))
During training, the fit
method will optimize the weights and biases of the neural network to minimize the loss function on the training data. After training, you can evaluate the model on new data using the evaluate
method:
loss, accuracy = model.evaluate(x_test, y_test)
print("Test loss: {:.4f}, accuracy: {:.4f}".format(loss, accuracy))
Comparing RNNs, GRUs, and LSTMs
In this example, we’re using the imdb
dataset from Keras, which contains 25,000 movie reviews labeled as positive or negative. We’re using the load_data
function to load the dataset, and we’re setting num_words
to 10,000 to keep only the 10,000 most frequent words. We’re pre-processing the data by padding the sequences to a fixed length of 100.
import tensorflow as tf
from keras.datasets import imdb
from keras.layers import Dense, Embedding, GRU, LSTM, SimpleRNN
from keras.models import Sequential
from keras.utils import pad_sequences
# Load the dataset
(x_train, y_train), (x_test, y_test) = imdb.load_data(num_words=10000)
# Preprocess the data
max_seq_length = 100
x_train = pad_sequences(x_train, maxlen=max_seq_length)
x_test = pad_sequences(x_test, maxlen=max_seq_length)
The example code defines three different models for sentiment analysis using RNNs, GRUs, and LSTMs, respectively. Let’s take a closer look at each of the models and their components:
# Define the RNN model
def create_rnn_model():
model = Sequential()
model.add(Embedding(10000, 32))
model.add(SimpleRNN(32))
model.add(Dense(1, activation='sigmoid'))
return model
The RNN model consists of an embedding layer, a SimpleRNN layer, and a dense layer with a sigmoid activation function. The embedding layer takes the input sequences and maps each word to a high-dimensional vector. The SimpleRNN layer processes the sequence of input vectors and maintains a hidden state that represents the context of the sequence. The dense layer produces a single output value between 0 and 1, which represents the predicted sentiment of the input sequence.
# Define the GRU model
def create_gru_model():
model = Sequential()
model.add(Embedding(10000, 32))
model.add(GRU(32))
model.add(Dense(1, activation='sigmoid'))
return model
The GRU model is similar to the RNN model, but it uses a gated recurrent unit (GRU) layer instead of a SimpleRNN layer. The GRU layer has additional gating mechanisms that allow it to selectively remember or forget certain pieces of information. This can help the model avoid the vanishing gradient problem that can occur in traditional RNNs, and improve its ability to model long-term dependencies in the data.
# Define the LSTM model
def create_lstm_model():
model = Sequential()
model.add(Embedding(10000, 32))
model.add(LSTM(32))
model.add(Dense(1, activation='sigmoid'))
return model
The LSTM model is also similar to the RNN model, but it uses a long short-term memory (LSTM) layer instead of a SimpleRNN layer. The LSTM layer has additional memory cells and gating mechanisms that allow it to selectively update its internal state based on the input data and the current state. This can help the model handle longer-term dependencies in the data and avoid the vanishing gradient problem that can occur in traditional RNNs.
Overall, these three models represent different ways to process sequential data and capture dependencies between different elements in the sequence. They can be trained on text data to perform sentiment analysis, or on other types of sequential data to perform a wide range of tasks such as time series forecasting, speech recognition, or music generation.
# Train the models
rnn_model = create_rnn_model()
rnn_model.compile(loss='binary_crossentropy', optimizer='rmsprop', metrics=['accuracy'])
rnn_history = rnn_model.fit(x_train, y_train, epochs=10, batch_size=128, validation_split=0.2)
gru_model = create_gru_model()
gru_model.compile(loss='binary_crossentropy', optimizer='rmsprop', metrics=['accuracy'])
gru_history = gru_model.fit(x_train, y_train, epochs=10, batch_size=128, validation_split=0.2)
lstm_model = create_lstm_model()
lstm_model.compile(loss='binary_crossentropy', optimizer='rmsprop', metrics=['accuracy'])
lstm_history = lstm_model.fit(x_train, y_train, epochs=10, batch_size=128, validation_split=0.2)
We’re compiling the model with the RMSprop optimizer, binary cross-entropy loss, and accuracy metric. Then, we’re training the model on our training data for 10 epochs, with a batch size of 128 and a validation split of 0.2.
Here’s an example of how you can evaluate the RNN, GRU, and LSTM models using the test data:
# Evaluate the models on the test data
rnn_loss, rnn_acc = rnn_model.evaluate(x_test, decoder_targets_test)
print("RNN model test loss: {:.4f}, accuracy: {:.4f}".format(rnn_loss, rnn_acc))
gru_loss, gru_acc = gru_model.evaluate(x_test, decoder_targets_test)
print("GRU model test loss: {:.4f}, accuracy: {:.4f}".format(gru_loss, gru_acc))
lstm_loss, lstm_acc = lstm_model.evaluate(x_test, decoder_targets_test)
print("LSTM model test loss: {:.4f}, accuracy: {:.4f}".format(lstm_loss, lstm_acc))
# Output
782/782 [==============================] - 8s 11ms/step - loss: 0.6919 - accuracy: 0.8100
RNN model test loss: 0.6919, accuracy: 0.8100
782/782 [==============================] - 4s 5ms/step - loss: 0.4834 - accuracy: 0.8272
GRU model test loss: 0.4834, accuracy: 0.8272
782/782 [==============================] - 3s 4ms/step - loss: 0.4337 - accuracy: 0.8390
LSTM model test loss: 0.4337, accuracy: 0.8390
We can see that the LSTM model achieved the highest accuracy on the test data, followed by the GRU model and then the RNN model. Here’s a breakdown of each model’s performance:
- RNN model: The RNN model achieved a test accuracy of 0.8100, with a test loss of 0.6919. The RNN model is the simplest of the three models, with the fewest number of parameters. This could explain its lower accuracy compared to the other models, as it may not have the capacity to learn more complex patterns in the data.
- GRU model: The GRU model achieved a test accuracy of 0.8272, with a test loss of 0.4834. The GRU model is more complex than the RNN model, as it uses gates to control the flow of information within the network. This additional complexity appears to have helped the model achieve a higher accuracy on the test data.
- LSTM model: The LSTM model achieved the highest test accuracy of the three models, with a score of 0.8390, and a test loss of 0.4337. The LSTM model is the most complex of the three models, with additional memory cells and gating mechanisms. This increased complexity appears to have allowed the model to learn more complex patterns in the data and achieve the highest accuracy.
It’s worth noting that the difference in accuracy between the models is relatively small, and could be affected by a number of factors such as the choice of hyperparameters, the size of the dataset, and the specific data samples in the test set. Overall, the results suggest that the LSTM model is the best performing model on this particular dataset, but further experimentation and analysis may be required to confirm this.
Here’s an example of how you can plot the training and validation loss and accuracy for the RNN, GRU, and LSTM models:
import matplotlib.pyplot as plt
# Plot the training and validation loss
plt.plot(rnn_history.history['loss'], label='RNN training loss')
plt.plot(gru_history.history['loss'], label='GRU training loss')
plt.plot(lstm_history.history['loss'], label='LSTM training loss')
plt.plot(rnn_history.history['val_loss'], label='RNN validation loss')
plt.plot(gru_history.history['val_loss'], label='GRU validation loss')
plt.plot(lstm_history.history['val_loss'], label='LSTM validation loss')
plt.title('Training and validation loss')
plt.xlabel('Epoch')
plt.ylabel('Loss')
plt.legend()
plt.show()
# Plot the training and validation accuracy
plt.plot(rnn_history.history['accuracy'], label='RNN training accuracy')
plt.plot(gru_history.history['accuracy'], label='GRU training accuracy')
plt.plot(lstm_history.history['accuracy'], label='LSTM training accuracy')
plt.plot(rnn_history.history['val_accuracy'], label='RNN validation accuracy')
plt.plot(gru_history.history['val_accuracy'], label='GRU validation accuracy')
plt.plot(lstm_history.history['val_accuracy'], label='LSTM validation accuracy')
plt.title('Training and validation accuracy')
plt.xlabel('Epoch')
plt.ylabel('Accuracy')
plt.legend()
plt.show()
This code will plot two graphs: one for the training and validation loss, and another for the training and validation accuracy. Each graph will show three lines representing the RNN, GRU, and LSTM models.
Note that we’re using the training history objects (rnn_history
, gru_history
, and lstm_history
) to access the loss and accuracy values for each epoch. We’re also using the plot
method from the matplotlib.pyplot
module to plot the graphs. You can adjust the graph titles, axis labels, and other parameters as needed.
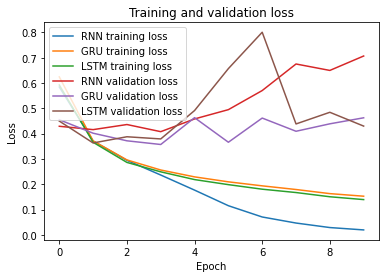
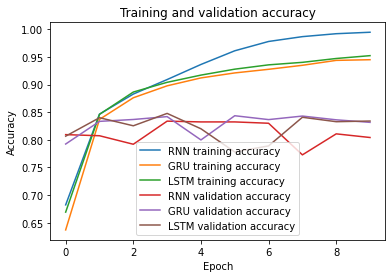
Further readings
Here are some technical resources for further reading about RNNs, GRUs, and LSTMs:
- Recurrent Neural Networks (RNNs):
- A Beginner’s Guide to Recurrent Neural Networks and LSTMs – A beginner-friendly guide to RNNs and LSTMs with code examples in Python.
- Recurrent Neural Networks – A technical overview of RNNs and their architecture, with mathematical formulas and visualizations.
- CS231n: Recurrent Neural Networks and Language Models – Lecture slides from a course on deep learning, with a section on RNNs and language models.
- Gated Recurrent Units (GRUs):
- On the Properties of Neural Machine Translation: Encoder-Decoder Approaches – The original paper proposing GRUs and comparing them to other RNN models for machine translation tasks.
- Learning Phrase Representations using RNN Encoder-Decoder for Statistical Machine Translation – Another seminal paper on GRUs, proposing an encoder-decoder framework for machine translation.
- CS224n: Natural Language Processing with Deep Learning – Lecture notes from a course on natural language processing, with a section on GRUs.
- Long Short-Term Memory (LSTM) Networks:
- Long Short-Term Memory – The original paper introducing LSTMs and describing their architecture and properties.
- Understanding LSTM Networks – A popular blog post with helpful visualizations and explanations of LSTMs and how they work.
- CS231n: Convolutional Neural Networks for Visual Recognition – Lecture notes from a course on deep learning, with a section on LSTMs.
These resources should provide a more technical and in-depth understanding of RNNs, GRUs, and LSTMs, and their applications in deep learning and artificial intelligence.